GUIs with pygame — wiki
From time to time, questions about GUI elements for pygame come up. The following sections give some links to GUI modules and libraries written for pygame and try - where possible - to give advice to which library you should refer for your pygame project.
A quick note to those who developed their own GUI system for Pygame: You are encouraged to add it to this page. Simply add a description, preferably in the form that the already existing ones provide, at the bottom of this page.
Which GUI type are you¶
Before you just use the first GUI library you find on the internet, you should ask yourself these questions:
- How much GUI do I need?
- How special are my requirements on GUI elements?
- What type of project am I working on?
Although the last question might sound a bit like an insult, it is possibly the most important question for your GUI decision. People (especially newcomers to game programming) tend to forget about their goal and, which is quite more important, the impression the project will have on its users.
Arcade games such as a Space Invaders project mostly do not need many GUI elements. It may need a text entry for the highscores and maybe some button basics for the main game menu. In contrast, a full featured economy simulation game like the good old Oil Imperium would need text entry boxes, buttons, lists for statistical data, table-like elements, spinner buttons for money amounts and many, many more.
Summing up those both fictional cases, the first one would need around two GUI elements while the second would need more than five. Additionally, if the arcade game has ingame GUI elements, those need to have a low reaction latency, while the economy simulation is mostly in the same gaming state showing an only slowly changing game window.
The user perspective¶
Another issue you have to deal with is the user perspective. The user perspective is a kind of usability from the user point of view and - in this case - simply means: "How much effort do I need to invest to run this game?". Far too often this question is silently ignored by newcomers, thus it is brought up here. If a user first has to install dependency X for the GUI you have integrated, then has to get and install the GUI and then can run the game, it is possibly too late (unless your game has outstanding concept/graphics/gameplay). They simply will remove it from their computer.
You therefore should check if the GUI library you are about to integrate in your game can be incorporated in your game distribution or if it has many third party dependencies, for which the integration/installation effort is higher than the benefit.
First of all, pygame relies on the SDL, which means that it can only have one window at a time. Thus, trying to implement multiple Gtk, Qt, ... application instances that use pygame, is an impossibility. The second problematic reason is that those toolkits use their own main loop, which possibly forces you to pipe their events to your pygame instance and vice versa. And to mention some other points in short: Drawing the toolkit elements on the pygame window is impossible and the SDL/pygame fullscreen mode will be problematic.
As you see, using those toolkits together with pygame will, in nearly any case, cause more problems than their usage solves. So let's quickly go to the next section.
GUI libraries¶
Now that you clarified those questions, you should know enough about the requirements you have on the specific GUI library type you want. The following presents a (not always up to date and possibly always incomplete) list of GUI libraries and modules suitable for the use with pygame. The entries also mention the basic capabilities and constraints the particular library has.
There is also a GUI comparision (2005) available, which was made by David Keeney for his own pygame project.
- thorpy
-
ThorPy is a GUI library for pygame that is pip install-able with excellent documentation.
- pgu
-
- OcempGUI (unmaintained)
-
Specialized GUI library with a rich GUI element set and a high functionality. It serves nearly all needs expected from a GUI library, which includes a relatively high (internal) complexity. Very suitable for a medium to high and very high GUI amount, a low GUI amount should be realized with another library, because OcempGUI would be overkill here. The incorporation into own distributions is possible, but more complex than with pgu, yet easy to integrate into existing code. As of version 0.1.1, includes internal support for Twisted, making it suitable for network games as well. Due to its high internal complexity the GUI element response time is somewhat lower than with other libraries, so that it should not be used in arcade like games or games, that have a real-time approach, criar sites.
Because of its rich feature set, it serves most needs of round based game types, simulation games, network games, or non-game applications.
- rich GUI element set based on pygame.
- high abstraction layers, own GUI elements can be created with less effort
- various accessibility (a11y) support layers reaching from full keyboard navigation support a system integration via the ATK/AT-SPI standards
- library supports own drawing engines and themes for user-defined look-and-feel
- includes internal support for Twisted via the TwistedRenderer
- easy to incorporate
- library is currently in a beta state, but very stable
- very suitable for round based and simulation games or applications
- full z-axis support using layers
- minimum dependencies are python and pygame only
- PyGVisuals
-
General GUI open-source-library. It provides most basic GUI-widgets (buttons, entries, labels, borders ...) while also offering some advanced widgets (e.g. for displaying lists). API-wise the library uses a object-orientated approach as all the widgets use pygame's Sprite-class. A widget's properties are controlled by its constructor and can be changed fully by calling the appropriate methods. Currently suitable for a low/medium GUI amount (e.g. arcade-like games, menues). More complex GUI's should be realized with another library as PyGVisuals is currently lacking a layout-manager.
- High abstraction widget-classes based on Pygame-Sprites, custom widgets can be easily added.
- Highly customizable widgets; widgets's properties can be changed while running the program.
- All basic widgets, some advanced widgets included.
- Feature requests, issues and feedback can be published via GitHub.
- Supports both python 2 and 3; pygame 1 and 2 will work both.
- Open-sourced: anybody can contribute to PyGVisuals, making it more stable and adding to its features.
- BSD-license: contents of this library can be used to one's liking as long as one follows the license's terms and conditions.
- Minimum dependencies are python and pygame only.
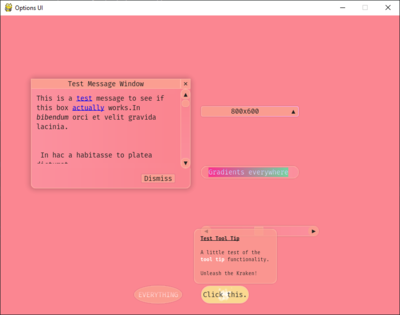
New in 2019, this GUI module aims for a modern appearance as well as being oriented directly towards games, with focused GUI elements like health bars alongside the standard buttons, sliders and drop down menus. The module also has approachable online documentation available here.
Features (Version 0.4.0)- Pygame 2 features & support.
- Extensive theming options for all elements, including:
- 'live theming' of your game, changing elements colours & appearance while the program is running.
- Different shapes for UI Elements including rounded rectangles & ellipses.
- Support for colouring elements with gradients as well as regular colours and all with semi-transparency if you like.
- HTML styling for text in the Text Box element, allowing for rich text display.
- Effects for Text Boxes; allowing for fades and the classic RPG-style 'typing effect'.
- Windowing system allowing for a screen full of GUI windows inside your pygame application.
- Uses Pygame classes and structures wherever possible such as sprites & events.
- Easy to install from PyPi with:
pip install pygame_gui
- Open source, no dependencies except Pygame, permissive MIT license.
- Open to collaborators, pull requests or feedback! The library is under active development, if you don't like something or want a feature that's not there, let us know on the issue tracker.